两年前我用C语言穷举法完成了,给老师检查,老师无情嘲笑了我的代码,今天做LeetCode又碰到了它。
虽然这次写得很烂,还是记录一下。
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98
| import java.util.ArrayList; import java.util.List;
public class Queen { public static void main(String[] args) { int n = 5; List<List<String>> answer = solveNQueens(n); System.out.println(answer); }
public static List<List<String>> solveNQueens(int n) { List<List<String>> bord = initBord(n); List<List<String>> answer = new ArrayList<>(); start(n,bord,answer,0);
return answer; }
public static void start(int n, List<List<String>> bord,List<List<String>> answer, int row){ if (row == n) { List<String> temp = new ArrayList<>(); for(int i = 0; i < n; i ++){ List<String> nowRow = bord.get(i); StringBuilder answerRow = new StringBuilder(); for(int j = 0; j < n; j ++){ if(nowRow.get(j).equals("Q")){ answerRow.append("Q"); }else{ answerRow.append("."); } } temp.add(String.valueOf(answerRow)); } answer.add(temp); return; } List<String> nowRow = bord.get(row); for (int col = 0;col < n; ++col) { if (check (row, col, bord, n)) { setOne(row,col,"Q",bord); start(n, bord, answer,row+1); setOne(row,col,"0",bord); }
} }
public static List<List<String>> initBord(int n){ List<List<String>> bord = new ArrayList<>(); for(int i = 0; i < n; i++){ List<String> temp = new ArrayList<>(); for(int j = 0; j < n; j++){ temp.add("0"); } bord.add(temp); } return bord; }
public static void setOne(int x,int y, String s, List<List<String>> bord){ List<String> row = bord.get(x); row.set(y,s); bord.set(x,row); }
public static boolean check(int x,int y, List<List<String>> bord,int n){ List<String> row = bord.get(x); for(int i = 0; i < n; i++){ if(row.get(i).equals("Q")) { return false; } }
for(int i = 0; i < n; i ++){ List<String> row1 = bord.get(i); if(row1.get(y).equals("Q")){ return false; } } for(int i = 0; i < n; i++){ for(int j = 0; j < n; j++){ List<String> row2 = bord.get(i); if((Math.abs(i-x) == Math.abs(j-y))&&(row2.get(j).equals("Q"))){ return false; } } } return true;
}
}
|
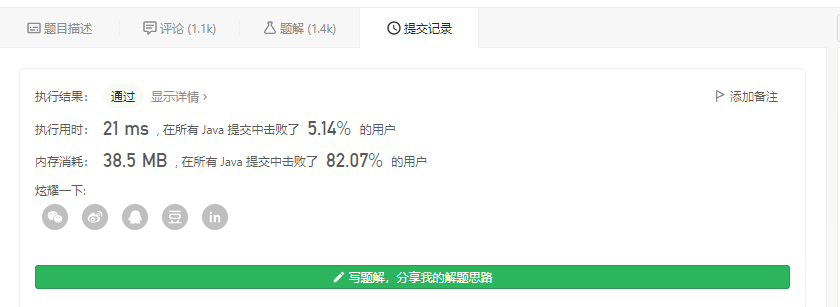